10. MAUI
MAUI is Microsoft's cross platform framework, it is the successor of Xamarin.Forms. It runs on Windows, Mac, iOS and Android, unfortunately not on Linux.
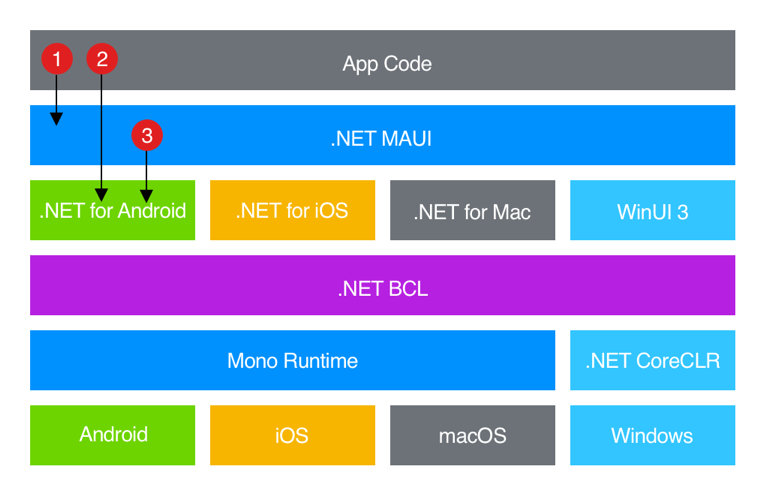
"In a .NET MAUI app, you write code that primarily interacts with the .NET MAUI API (1). .NET MAUI then directly consumes the native platform APIs (3). In addition, app code may directly exercise platform APIs (2), if required.
.NET MAUI apps can be written on PC or Mac, and compile into native app packages:
- Android apps built using .NET MAUI compile from C# into an intermediate language (IL) which is then just-in-time (JIT) compiled to a native assembly when the app launches.
- iOS apps built using .NET MAUI are fully ahead-of-time (AOT) compiled from C# into native ARM assembly code.
- macOS apps built using .NET MAUI use Mac Catalyst, a solution from Apple that brings your iOS app built with UIKit to the desktop, and augments it with additional AppKit and platform APIs as required.
- Windows apps built using .NET MAUI use Windows UI 3 (WinUI 3) library to create native apps that target the Windows desktop. For more information about WinUI 3."1
The following diagram shows an overview of the .NET MAUI app lifecycle:
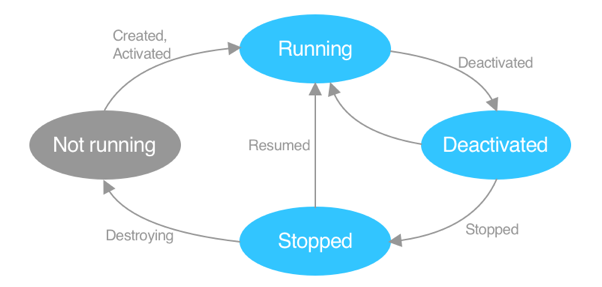
10.1 Getting started
- MacOS Environment Setup for MAUI Development with Rider
- Windows Environment Setup for MAUI Development with Visual Studio Community -- Rider does not support hot reload on Windows. If you want to build Windows Application with MAUI, use Visual Studio instead of Rider.
- Linux Environment Setup for MAUI Development with VS Code
First App
Create a new solution based on the template MAUI, run it and try to understand the code.
Check out .Net MAUI Architecture Overview to understand, how everything is clued together.
10.2 UI
Here you find all built-in controls. There is no visual designer and no preview in the IDE. But, Rider on Mac seems to support hot reload on iphone emulators and Visual Studio Community on Windows supports hot reload for Android emulators.
10.3 Navigation
For mobile apps you need navigation. The visual hierarchy is defined in xml, AppShell.xaml.
Simple Navigation
For a good introduction watch Navigating Between Pages in .NET MAUI [6 of 8] | .NET MAUI for Beginners.
The following code defines the starting pages, mobile this will be shown as a bottom navigation, on the desktop as tabs.
<TabBar>
<ShellContent Title="Main"
Icon="home.svg"
ContentTemplate="{DataTemplate pages:MainPage}" />
<ShellContent Title="Students"
Icon="university.svg"
ContentTemplate="{DataTemplate pages:StudentsPage}" />
</TabBar>
To define a navigation drawer/flyout navigation on mobile use the following code.
<FlyoutItem Title="Main">
<ShellContent ContentTemplate="{DataTemplate pages:MainPage}"/>
</FlyoutItem>
<FlyoutItem Title="Students" >
<ShellContent ContentTemplate="{DataTemplate pages:StudentsPage}"/>
</FlyoutItem>
And to use the Flyout for desktop+tablet and the tabbar for mobile only define both as above and
1. Add in XAML <Shell ... FlyoutBehavior="{OnIdiom Phone=Disabled, Default=Locked}"...
2. Add a name to your TabBar <TabBar x:Name="PhoneTabs">
3. In your App.xaml.cs add
public AppShell()
{
InitializeComponent();
if (DeviceInfo.Idiom != DeviceIdiom.Phone)
Shell.SetTabBarIsVisible(this, false);
}
Sub/details pages should be defined in AppShell after InitializeComponent, e.g.
Routing.RegisterRoute("students/demo", typeof(DemoPage));
To navigate to this page from the students page use await Shell.Current.GoToAsync("demo");
.
And parameters may be passed as query parameters, e.g. await Shell.Current.GoToAsync("demo?name=xxx");
and the receiving part of the parameter looks like
[QueryProperty(nameof(Name), "name")]
public partial class DemoPage : ContentPage
{
string _name;
public string Name
{
get => _name;
set
{
_name = value;
OnPropertyChanged();
}
}
...
}
// or with a ViewModel
public class StudentDetailViewModel : IQueryAttributable, INotifyPropertyChanged
{
public string Name { get; private set; }
public void ApplyQueryAttributes(IDictionary<string, object> query)
{
Name = query["name"] as string;
OnPropertyChanged("Name");
}
...
}
10.4 MVVM

To write less boilerplate code it is suggested to use the nuget package MVVM Toolkit. MVVM Toolkit Features gives you a good introduction into MVVM (repetition from what you know of working with MVVM in Avalonia) and how to use the toolkit in MAUI. The figure above shows you the weather sample app, analyze the source code to get an idea how typical problems are solved in MAUI.
10.4.1 Learning Path
- Simple Notes App - about 50min
- Upgrade your app with MVVM concepts - about 1h
- .NET MAUI - Workshop - about 4h just the video walkthrough
10.5 Access REST
The best way to access remote data is to implement a service, see Accessing remote data and the complete app source code eshop-mobile-client.
10.6 Putting things together
Read the article Putting things together how to put REST, MVVM ... together and build a very simple and minimalistic Reddit browser for a select number of subreddits.
10.7 Further Reading
- Architecture Guide -- an example of a enterprise application with backend and different clients.
- Icons: I suggest to use the package MauiIcons to display icons in your app. Alternatively, you could use font items as described Display font icons and
How to Use Material Design Icons In .NET MAUI.
- Localization maybe implemented easily following the instructions provided by Microsoft, Localization.
- Add an app icon to a .NET MAUI app project.
- Theme an app.
- Data binding and MVVM.
- Build mobile and desktop apps with .NET MAUI provides a very good introduction into .Net MAUI including how to consume a REST service.
- Official .NET MAUI Samples
- .NET MAUI Samples
-
David Britch. What is .net maui? - .net maui. 2023. URL: https://learn.microsoft.com/en-gb/dotnet/maui/what-is-maui?view=net-maui-8.0 (visited on 05.03.2024). ↩